React introduces a component model to compose your UI. There are a couple of ways of rendering a component/JSX.
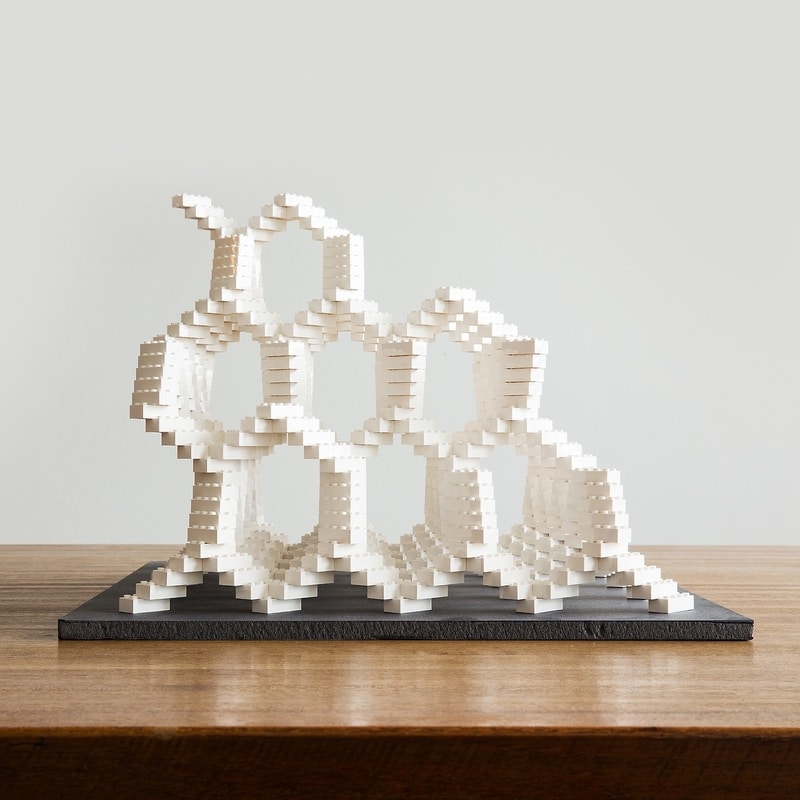
React introduces a component model to compose your UI. There are a couple of ways of rendering a component/JSX. You can use these simples rules to determine which way to write your component.
Note: This is a follow up article based on youtube screencast I made. check it out if you prefer watching. https://youtu.be/tIK8ndsC4SE
The ways
It's possible to write components using classes or functions. When using classes what we do is extend either React's Component or PureComponent.
Both Component and PureComponent gives us access to React's state handler and lifecycles. The difference between both is PureComponent implements the shouldComponentUpdate lifecycle.Components can also be any type of function as long as it returns a valid React Node.
When using functions it's more common to use arrow functions assigned to a const variable.
Determine which to use
We will use class components every time we need to use either lifecycles and/or state. That's why function components are regarded as Stateless Functional Components or Dumb Components.Second, when we need a re-rendering aware component we should use a PureComponent or implement a class component with shouldComponentUpdate. Components can re-render every time props change, however, we might not always need that re-render. A common scenario is when the parent and a sibling component updates, this can cause a re-render. To avoid this you can leverage shouldComponentUpdate or just extend the PureComponent.For every other case your component should be a Stateless Functional Component, it renders faster. With functional components you can use them in two ways. You can declare them in JSX as a regular component or call it as a function.
Both FunctionComponent and functionalComponentTwo could be written the same way, just remembering how you would access props, as a JSX component the props are passed as an object to the first argument of the FunctionComponent, while calling a function you would pass your props as arguments to the function then access it as usual.
This article by Peter does a benchmark between Class Components, Function Components, and regular Functions. A regular function is the fastest, however, it's just 1ms faster which would not really impact the overall performance. Since a Function Component gets instantiated as a class component behind the scenes, it's just 14% faster than a class component, React's core team have stated their intentions to optimise Function Components, although there has been no plan to when.
To keep consistency always use your Stateless Function Components as JSX components instead of functions in JSX. But here is a scenario where it might be interesting to simply call a function inside JSX. When breaking down the render method, in cases where your JSX tree can be broken down into parts, you can do as follow.
If you aren't accessing values from the either the state or props you don't need to instantiate it within the class.
Another case where we see a function being called inside JSX is when using a Function as Child Component pattern, however I won't cover this on this post. Here is a link if you want to learn about it. Thanks for reading, I’m Andrei Calazans a Software Developer at G2i who is in love with learning and teaching. Feel free to reach out and chat.
https://github.com/AndreiCalazans
https://twitter.com/Andrei_Calazans